As C# is still a key language used to build powerful applications both inside the .NET Framework and beyond, it is natural that C# developers would want to load weather data into their applications. Luckily, C#’s built-in functions make it easy to compose and execute web queries and also easy parse the JSON results into objects that can be used directly within any C# application. In this article we’ll show you how to load and use weather data within your own C# applications.
This guide assumes that you already have a Visual Crossing Weather account. If you don’t already have one, however, it is free and easy to sign up. Simply provide your email address, obtain your API key, and you will be ready to follow the steps below to load weather data using C#.
Building a weather query URL
The first step in loading weather data into your C# code is to create a weather query URL. You can do this in various ways including by using our web-based query page. Simply define your query in the interface, and the page will generate the query URL for you automatically. Alternately, the weather query can be constructed either manually or in code by following the guidelines in the Weather API documentation.
One of the easiest weather queries and the one that we will use in the remainder of this guide is a simple Timeline Weather query for the 15-day forecast. In the URL for this query, we only need to specify the target location. The weather engine will infer that we wish to query the forecast. That query URL takes this form:
https://weather.visualcrossing.com/VisualCrossingWebServices/rest/services/timeline/<LOCATION>?key=<YOUR_API_KEY>
Simply replace the <LOCATION> placeholder with your desired target location, for example, Paris, France and the <YOUR_API_KEY> with the API Key for your account. If my API Key is 1234567890, I could use the follow query to obtain the 15-day forecast for Paris.
https://weather.visualcrossing.com/VisualCrossingWebServices/rest/services/timeline/Paris?key=1234567890
Of course, this query is the most basic weather query example. I could make it more sophisticated by changing it to query any location on any date, past, present, or future. You can learn more about the Timeline Weather API and the available options and parameters in our documentation. It is good to get familiar with the API options because you will often want to build query URLs in your C# code instead of running hard-coded URLs. Using the documentation, you can assemble the URL parameters easily to create all types of custom and dynamic queries.
One key benefit of a URL API is that you can test the query URL in any browser and instantly see the JSON result. The Paris query example above should produce a result that looks like this.
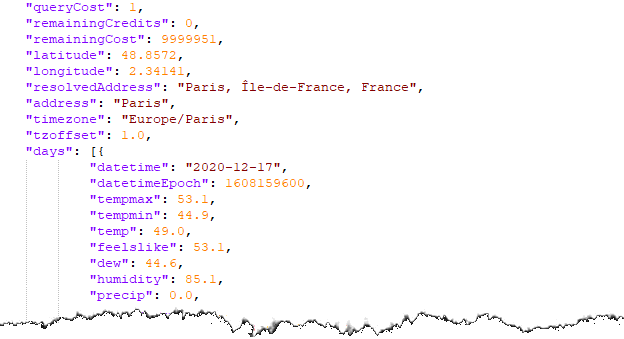
Executing a query URL in C#
Once you have a weather query URL either created manually, via the weather query page, or constructed in your own C# code, you are ready to run this query. C# has several different ways to query a URL including the popular RestSharp library. However, in this article we will use the more traditional HttpClient calls to do this work. In this example, we will use the entire query URL as a single, static string. However, more sophisticated code would use the C# code to actually construct the the URL from pieces and parameters.
We can then use HttpClient’s SendAsync() to send the query URL to the weather server and ReadAsStringAsync() to retrieve the response data. C# will automatically handle the network communications between our client and the weather server, and it will return to us the HTTP response. From that response we can retrieve the HTTP response body, and that body will contain our weather result data.
Plese find below an example code snippet that shows the necessary steps.
var client = new HttpClient(); var request = new HttpRequestMessage(HttpMethod.Get, " https://weather.visualcrossing.com/VisualCrossingWebServices/rest/services/timeline/Paris?key=<YOUR_API_KEY>"); var response = await client.SendAsync(request); response.EnsureSuccessStatusCode(); // Throw an exception if error var body = await response.ReadAsStringAsync();
Parsing JSON weather results in C#
Now that we have the weather data available to us as delivered in the query response body, our final step before we can use the data is to parse it as JSON. As with the task of querying a URL, there are several ways to parse JSON in C#. One of the most common is to use Json.NET, so that is the method we will use in this example. However, you should be aware that there are other options available including Json in System.Web.Helpers among various other options.
To show how to deserialize the weather JSON, we’ll pick up the sample code above from where we left off. A single call to JsonConvert.DeserializeObject() will take the weather result data and convert it into a .NET object. Our C# code can then read the weather values from the object and use them anywhere. For example, we could take the condition description, temperatures, and rainfall chance to produce a weather display on a webpage or in a dashboard. Alternately, our code could iterate through all of the available measures and feed the dataset into a database or to a custom data analysis engine. Once the weather data is available in an object, its use is limited only by your C# code. However, in this simple example code, we’ll settle for printing a few key measures for each day in the forecast.
var body = await result.Content.ReadAsStringAsync(); // From the URL query code above dynamic weather = JsonConvert.DeserializeObject(); // Loop through every "day" in the JSON and print a few details foreach(var day in weather.days) { string weather_date = day.datetime; string weather_desc = day.description; string weather_tmax = day.tempmax; string weather_tmin = day.tempmin; Console.WriteLine("Forecast for date: " + weather_date); Console.WriteLine(" General conditions: " + weather_desc); Console.WriteLine(" The high temperature will be " + weather_tmax); Console.WriteLine(" The low temperature will be: " + weather_tmin); }
Summary
In this article you have seen how easy it is to create a weather query using Visual Crossing Weather, run that query in C#, and then parse the JSON results. Once you have these parsed results, you are ready to use the data in your own weather use case. Since C# is often used to build web applications and and in complex data analysis cases, your next step might be to feed weather data into a web display or make an analysis application comparing business data to weather results over time.
Of course, the forecast query example in this article shows only the most basic example of how to use the Visual Crossing Weather API in C#. However, even from this simple query, you can read many different weather forecast measures including current conditions. The Timeline Weather API also supports historical queries that can return weather reports from any global location for any time and date since 1970. Beyond that, our statistic (climate-based) forecasts allow you to see weather norms for any date of the entire year. When coding in C#, you will be able to put this functionality and much more to work in your weather-enabled applications.
Questions or need help?
If you have a question or need help, please post on our actively monitored forum for the fastest replies. You can also contact our Support Team.